How to Upload Url on Perfect Player
Here I am going to show y'all how to upload and play video using Django framework in Python programming. The uploaded video may or may non be played automatically merely the uploaded video will have controls, such as, paly, pause, full screen, mute, unmute, download, etc.
An upload form is displayed to the cease user for browsing and selecting a video file that volition be uploaded and displayed on the browser. I am using HTML5 tag to play the video. Make sure your browser supports HTML5 tag for playing video.
Prerequisites
Python: iii.9.5, Django: 3.2.iv
Projection Setup
Yous generally create a Django projection anywhere on your system and under your Django projection you have one or more than applications.
Let'southward say you have created Django project directory calleddjangovideouploaddisplay and you lot have besides created an app calledvideouploaddisplay underdjangovideouploaddisplay. Yous may cheque the documentation for creating Django projection and apps nether project.
I presume you have the required configurations for your videouploaddisplay in djangovideouploaddisplay/djangovideouploaddisplay/settings.py file underINSTALLED_APPS
section equally beneath:
INSTALLED_APPS = [ ... 'videouploaddisplay.apps.VideouploaddisplayConfig', ]
Thevideouploaddisplay.apps.VideouploaddisplayConfig
is formed as a dotted notation from djangovideouploaddisplay / videouploaddisplay /apps.py, where y'all will find the class name asVideouploaddisplayConfig
that has name videouploaddisplay .
In your settings file – djangovideouploaddisplay/djangovideouploaddisplay/settings.py, defineMEDIA_ROOT
andMEDIA_URL
, for example:
MEDIA_ROOT = os.path.join(BASE_DIR, '') MEDIA_URL = '/'
In the above configuration I am non specifying whatsoever directory for storing the video but the video will be uploaded in the root directory of the projection.

Template or View File
The template or view file is nothing but you write code on HTML file what you lot want to display on UI (User Interface). For this video file upload functionality I am going to put only one field that is file which volition be used for browsing and selecting file.
The proper name of the beneath template file isupload-display-video.html and put it nethervideouploaddisplay/templates directory.
<!DOCTYPE html> <html> <head> <title>Django - Video File Upload and Display</title> </head> <trunk> <div fashion="width: 500px; margin: auto;"> <fieldset proper name="Video File Upload and Display"> {% if msg %} {% autoescape off %} {{ msg }} {% endautoescape %} {% endif %} <class method="post" activity="/" enctype="multipart/form-data"> {% csrf_token %} <dl> <p> <label>Scan and select a video file</label> <input blazon="file" name="file" autocomplete="off" required> </p> </dl> <p> <input blazon="submit" value="Upload and Display"> </p> </form> </fieldset> {% if filename %} <div style="margin: 10px auto;"> <video autoplay="autoplay" controls="controls" preload="preload"> <source src="{{ MEDIA_URL }}/{{ filename }}" type="video/mp4"></source> </video> </div> {% endif %} </div> </body> </html>
In the above file user selects a video file and if file gets successfully uploaded into the root directory of the project folder and then beneath the upload grade I am displaying the video that will starts playing automatically.
The another important thing is you need to set up CSRF token on the form using{% csrf_token %}
otherwise you won't be able to upload file and you lot would go the following errors:
CSRF token is not set CSRF Token missing or incorrect
You lot as well demand to ensure to check the CSRF token on your server side code and you volition afterwards when you get through the code inviews.py file.
Form
The DjangoForm
will map the fields to course on your template file. In the above template or view file I take kept just one field calledfile
which is used to select a file. A view treatment this class will receive data intorequest.FILES
.
request.FILES
will only incorporate data if the HTTP asking method isPOST, at least one file is posted and the corresponding form has an attributeenctype="multipart/form-data"
, because dealing with forms that have FileField and ImageField fields are more than circuitous.
The following code snippets are written intoforms.py file under directoryvideouploaddisplay.
from django import forms class UploadFileForm(forms.Form): file = forms.FileField()
Views
In this view I am going to show how to upload file into a server location. You will also see how file data is passed fromrequest toForm
. Here also notice how I have used CSRF decorator to ensure the CSRF token.
from django.shortcuts import return from .forms import UploadFileForm from django.views.decorators.csrf import ensure_csrf_cookie @ensure_csrf_cookie def upload_display_video(request): if asking.method == 'Post': class = UploadFileForm(request.POST, request.FILES) if course.is_valid(): file = request.FILES['file'] #impress(file.name) handle_uploaded_file(file) return render(request, "upload-brandish-video.html", {'filename': file.name}) else: form = UploadFileForm() return render(request, 'upload-display-video.html', {'form': grade}) def handle_uploaded_file(f): with open up(f.name, 'wb+') as destination: for chunk in f.chunks(): destination.write(chunk)
Note that you have to pass request.FILES into theForm
'southward constructor to demark the file data into aForm
.
Looping overUploadedFile.chunks()
instead of usingread()
ensures that big files don't overwhelm your system'southward retentiveness.
Ideally the functionhandle_uploaded_file()
should be put into common utility file, only just for the sake of this example I have kept into the same filevideouploaddisplay/views.py.
Before you salvage the file onto disk the uploaded file data is stored under thetmp folder and the file proper name is generated something similartmpzfp312.upload. If the file size is upward to 2.5 MB then file is not saved nether thetmp folder and unabridged content is read from the retentiveness and the reading becomes very fast.
URLs
Now y'all demand to define the path or URL which will call the appropriate function (for this example,upload_display_video()
) on yourviews.py file. You need to create a fileurls.py nethervideouploaddisplay folder with the following code snippets.
from django.urls import path from django.conf import settings from . import views urlpatterns = [ path('', views.upload_display_video, name='upload_display_video'), ]
I want to upload and brandish video on root URL, and so I did not mention any path on the URL.
Thepath()
function is passed four arguments, 2 required:road andview, and two optional:kwargs, andname.
route is a cord that contains a URL pattern.
view calls the specified view function with an HttpRequest object as the first argument and any "captured" values from the route as keyword arguments.
kwargs are arbitrary keyword arguments can be passed in a dictionary to the target view.
name – naming your URL lets you refer to it unambiguously from elsewhere in Django, particularly from within templates.
Another of import matter you demand to practise is to configure the URL into the project. This (videouploaddisplay) is an app and your project does non know annihilation most this URL. So add the following line intodjangovideouploaddisplay/djangovideouploaddisplay/urls.py file underurlpatterns = [...]
.
path('', include('videouploaddisplay.urls')),
You demand to also include the MEDIA_URL which you defined earlier in settings.py file. The complete content for this djangovideouploaddisplay/djangovideouploaddisplay/urls.py file is given beneath:
#from django.contrib import admin from django.conf import settings from django.urls import path, include from django.conf.urls.static import static urlpatterns = [ #path('admin/', admin.site.urls), path('', include('videouploaddisplay.urls')), ] + static(settings.MEDIA_URL, document_root=settings.MEDIA_ROOT)
Deploying Application
Now I am prepare to test the app I have built. Let'due south fire up the server from the command line usingmanage.py runserver
. The application will run on default port8000
. If y'all want to change the default host/port of the server then you lot can read tutorial How to change default host/port in Django.
Testing the Application
When you striking URLhttp://localhost:8000 on browser, your abode page looks similar to the following image:

When you lot upload a video your video will be started playing automatically. Hovering the video will brandish the controls for video.
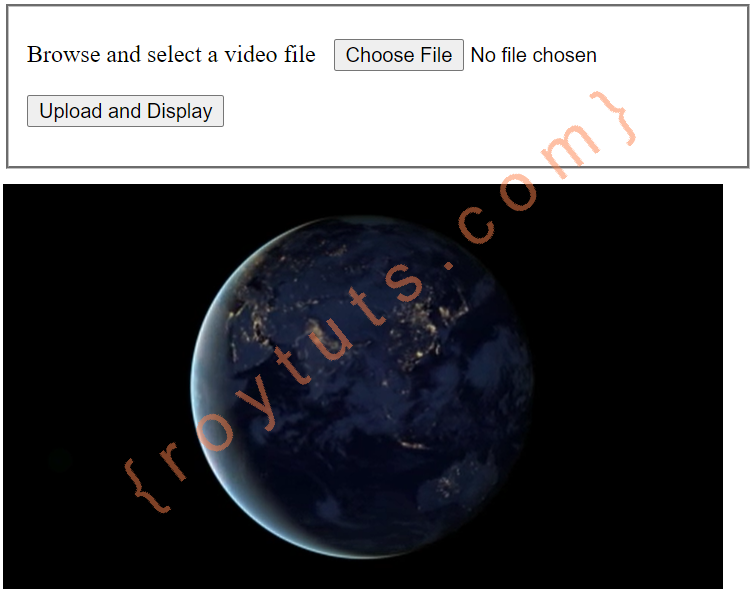
That'southward all about how to upload and play video using Django in Python.
Source Code
Download
Source: https://roytuts.com/upload-and-play-video-using-django/
0 Response to "How to Upload Url on Perfect Player"
Post a Comment